Querying for Latest Prompt
You can use the Izlo API to get the latest prompt text from any prompt stored within the Izlo database. This makes it easy for your applications to stay up to date with what is the most current prompt to be using. With the right setup, it can also be used as a way to empower prompt engineers to update prompts within Izlo that will automatically update within your application or website.
The latest prompt endpoint lives at: https://getizlo.com/api/v1/get-prompt/
You will also need to pass `prompt_id` to the API to pull the prompt you need. You can programmatically see a list of your prompts at our All Prompts Endpoint or you can copy the prompt id by finding the prompt within the application and clicking the copy icon.
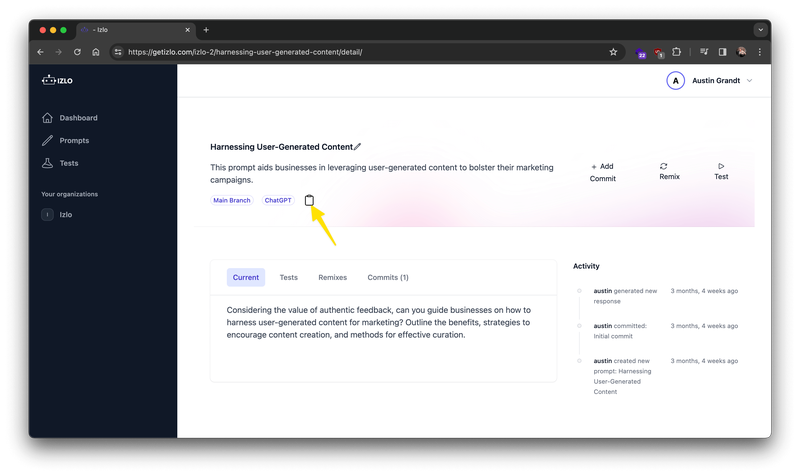
Once you have the ID of the prompt you want to pull you can make queries as follows.
let izloApikey = 'YOUR_API_KEY'
let izloPrompt = 'YOUR_PROMPT_ID'
let izloURL = 'https://getizlo.com/api/v1/get-prompt/?prompt_id=' + izloPrompt
fetch(izloURL, {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer ' + izloApikey,
},
})
import requests
izloPrompt = 'YOUR_PROMPT_ID'
url = 'https://getizlo.com/api/v1/get-prompt/?prompt_id=' + izloPrompt
token = 'YOUR_API_KEY'
headers = {
'Authorization': 'Bearer ' + token
}
response = requests.get(url, headers=headers)
The above code will get the specific prompt from your database. An example response might be:
{
"id": "PROMPT_ID",
"name": "Summarize Blog Posts",
"prompt_text": "You are a blog post writer who is tasked with summarizing a blog post. The blog post could have any topic, it is up to you to read it and understand it. You should use a professional voice to summarize this post into 4 distinct bullet points. An example of a response could look like:\r\n\r\n<ul>\r\n<li>This article discusses how to find happiness in a new job</li>\r\n<li>It also talks about how to find friends in the workplace</li>\r\n<li>There is also some discussion about how to find happiness at work in a remote setting</li>\r\n<li>It ends with some suggestions on getting started finding a new job</li>\r\n(generate more bullet points)\r\n</ul>\r\n\r\nUse the below text to return an HTML formatted bulleted list of the main 4 points from the below post. All bullet points should be succinct and should summarize the article below. Remember to only return and HTML unordered list:\r\n\r\n{# post_text #}\r\n\r\nYour Summary:",
"description": "This prompt will create a list of bullet points at the end of a blog post for the summary",
"created_at": "2023-07-11T12:37:52.207755-05:00",
"updated_at": "2023-07-11T12:37:52.228947-05:00"
}
Please note that your response will have any variables you have defined in the prompt text.